wizzwizz4
# [Python 2] REPL, ~~53~~ 40 bytes
while _>4:sum(int(x)**2for x in`_`)
_<2
[Try it online!][TIO-kiqik1w0]
## Explanation
The 37 cycle contains a 4. This means that all input values will eventually end up less than 5. Of the numbers `[1, 2, 3, 4]`, only `1` is a happy number; we know this, so we don't need to recurse any more. Checking for `1`, here, is equivalent to checking for `< 2`; the latter is shorter.
In Python 2, `_` is equivalent to `repr(_)`, which for integers is equivalent to `str(_)`. `**` is the “to the power of” operation in Python, and generators don't need to be enclosed by additional brackets if they're the only argument to a function. In the REPL (code compiled with the `'single'` mode), the value of the last statement is assigned to the `_` builtin.
This code assigns the value `False` for truthy and `True` for falsy – i.e., it answers the question in the title, not the question specified in the challenge body.
[Python 2]: https://docs.python.org/2/
[TIO-kiqik1w0]: https://tio.run/##nVJNb8IwDL33V1hIqA1j1UCTJiHojUm7bIftxlApxR0ZrROlQYRfz9zy/XWZL4md52fn6em1nSvqbjKjCkjVDEEWWhnL90LLHGM@i4RmXg2wJklxmqSLPUobSTZGl3reDDMwqPNAip4HHBIGIC0aLtQ5cf5U31ZzZoYvs8QtsoqaCfwoivz2oWjN@oiowjEHobN7zvNud1bLJWHJ@JEbH@q8KWoLn1bpN14tsVLR@YSpwWRxqNzatIqUeS8UCvxv8sNfJSmoJ4s2ZPxMSYEDvy9JL22zjPwmnW8uMyaTJZCy8K7oYs71RidahWF4otW/5AkTrZF3d@K@5ugwDVJxoWHvekDlg@CII3gYQGdrjMpZMSszxUwZbEO58whn7BNJYBL6wd2zOHKvJeYzflzFtYA79qrLVV1lWOpc2lp6Mer0Hjvjy15313FXH7097MQ2w4/XoTHK3HKMV3t//89OG0yj0dhs58bRc69cFgFrxEq3Wt39BybxRHhe3O9uGCzE5uUP "Python 2 – Try It Online"
Answer #2
xigoi
# [Jelly], 7 bytes
D²SƊÐL’
[Try it online!][TIO-khpb7etc]
[Jelly]: https://github.com/DennisMitchell/jelly
[TIO-khpb7etc]: https://tio.run/##y0rNyan8/9/l0KbgY12HJ/g8apj5/3D7o6Y1//9HG@ooGOkoGOsomOgomOoomOkomOsoWOgoWOooGBoAMVDeEKjAEKjC0CQWAA "Jelly – Try It Online"
Returns truthy (≠0) for happy numbers and falsy (0) for sad numbers.
## Explanation
D²SƊÐL’ Main monadic link
ÐL Repeat until the results are no longer unique
Ɗ (
D Decimal digits
² Square [each]
S Sum
Ɗ )
’ Decrement
Answer #3
Draco18s
# [Runic Enchantments], 60 bytes
Duqi/'^̖1}͍?X)1l+\
Rc4*-:0(4*?:*}!/
/:'%≠?@'/Squ
\?=1:+\
[Try it online!][TIO-knibi8gf]
[Runic Enchantments]: https://github.com/Draco18s/RunicEnchantments/tree/Console
[TIO-knibi8gf]: https://tio.run/##KyrNy0z@/9@ltDBTXz3uzDTD2rO99hGahjnaMVxBySZaulYGGiZa9lZatYr6XPpW6qq2Z3bYO6jrBxeWcsXY2xpaacf8/29oAAA "Runic Enchantments – Try It Online"
Still working on the path visualizer, but it's coming along pretty nicely.
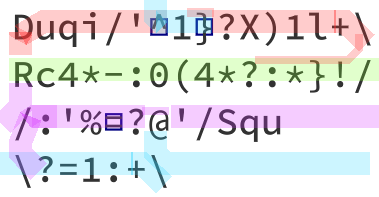
Visualizer displays unicode combining characters as boxes (Unity issue) and there's still folds in the line corners and the occasional path overlap that's hard to avoid (but I manually hid those). Bends when it switches from skip to not skip (top right) are also hard to fix, as are bends after wrapping.
Program starts at the `^̖` (top, blue) and while it initializes pointing up, the reflection modifier bounces it to the left (this is 1 byte shorter, due to the space available on the first line and where things line up on the last line--this is rare!).
Basically the program reads in a number, concats a `/` onto it, then parses it into a sequence of characters (top left), subtracts 48 from each and squares (green) using "value less than 0" as a loop terminator (loop exit the thin red bit). Then adds the stack back up again (top right), adds 1 (`/` is ascii 47, so the program add a -1 to the sum; popping and adding a zero didn't fit on the second line; bottom left), then checks for 1-ness and 37-ness (ascii `%`; bottom two, left). If not, it concats a new `/`, and re-enters the initial loop (third line, right).
If 1 or 37, print the entire stack (the only remaining value being a 1 or 37).
There's also a rare "not-equals" for checking for 37-ness, as boolean negation isn't very optimal (takes a lot of space), involves swapping the locations of the two halves of the logic, or `!` skipping if one of the sides is only a single instruction. The latter is true here, but the extra command, saving 1 byte, moves the `@` out of line to receive the 1-ness exit, so an extra `@` is needed.
Answer #4
Razetime
# [Husk], 6 bytes
←ω(ṁ□d
[Try it online!](https://tio.run/##yygtzv7//1HbhPOdGg93Nj6atjDl////hpbGAA "Husk – Try It Online") or [Verify first 99 values][TIO-km298wsn]
[Husk]: https://github.com/barbuz/Husk
[TIO-km298wsn]: https://tio.run/##AT0Awv9odXNr/20owqcrb2ArIiAtPiAic28/InRydWUiImZhbHNlIuKCgSnhuKP/4oaQz4ko4bmB4pahZP///zk5 "Husk – Try It Online"
## Explanation
```
←ω(ṁ□d
ω( repeat the following until fixpoint:
d get the digits
ṁ□ sum of their squares
← decrement