Given a string as an input (which can be any acceptable/convenient format in your language), implement pendulum encoding. The test cases are split into individual items (which aren't quoted) for a visually appealing explanation. ## How do I do that? The current **iteration index** starts at `0`. * If the iteration index is even, append the current item onto the output string. * If the iteration index is odd, prepend the current item onto the output string. ## An example ``` The input is [a b c d e f g]. Note that the letters a-g are individual one-character strings, to prevent confusion from the iteration index. N: the iteration index N:0 Out: [a] N:1 Out: [b a] N:2 Out: [b a c] N:3 Out: [d b a c] N:4 Out: [d b a c e] N:5 Out:[f d b a c e] N:6 Out:[f d b a c e g] ``` The output should be `[f d b a c e g]`. # Another example ``` The input is [u d l n u e m p]. N:0 Out: [u] N:1 Out: [d u] N:2 Out: [d u l] N:3 Out: [n d u l] N:4 Out: [n d u l u] N:5 Out: [e n d u l u] N:6 Out: [e n d u l u m] N:7 Out:[p e n d u l u m] ``` ## Test cases [Here](https://tio.run/##lVLNaoQwEL77FHNZMBhl22OXLeToZdmjyxJK6o5LQKONkV767naibbQUSp1LyMz3MzMJ2lqZe4p9aXXnxjHq0NyGemjiq@QgOLwwSJ9BHKJV5fJRzMXcFyOg2IFun96tdhgLxpeUqeOvq66mw0cOFht4hOMR9l59B6Lz6llA@Ah@BXlBksD1Qq45JPDADgHp7ICzxtni3yLE9zLiW2QCToxltiJMtGLSKvaMUI3ShvYSIGHkZTWKv/KS3zjyit8lY/znDn4T5Lpb@AdBya2EuSW5hSA3OyjyIB@5JmT0KqfWpIPRb/RKDnuXlqpHqGzb0B@gZO@ycfwE)'s a sample program doing this encoding. Take note that the characters in the string aren't always unique. ``` Your output *has* to be flattened. [a,b,c,d,e,f,g] -> [f,d,b,a,c,e,g] [] -> [] [a] -> [a] [a,b,c,d] -> [d,b,a,c] [a,b] -> [b,a] [a,b,d] -> [b,a,d] [a,b,a,c,b,c] -> [c,c,b,a,a,b] [a,a,b,b,c,c] -> [c,b,a,a,b,c] [u,d,l,n,u,e,m,p] -> [p,e,n,d,u,l,u,m]
# [APL (Dyalog Extended)], 11 [bytes](https://codegolf.meta.stackexchange.com/a/9429/43319 "When can APL characters be counted as 1 byte each?") ([SBCS](https://github.com/abrudz/SBCS ".dyalog files using a single byte character set")) ```apl -\`∘⍳∘≢⍋⍛⊇⊢ ``` [Try it online!][TIO-k88l7mbf] (Empty case works offline, but TIO hasn't been updated.) `-\` alternating sum `` `∘`` of `⍳` **ɩ**ndices `∘` of `≢` the length `⍋` graded (indices that would sort) `⍛` before `⊇` being used to select from `⊢` the argument [APL (Dyalog Extended)]: https://github.com/abrudz/dyalog-apl-extended [TIO-k88l7mbf]: https://tio.run/##SyzI0U2pTMzJT9dNrShJzUtJTfn/Xzcm4VHHjEe9m0Fk56JHvd2Pemc/6mp/1LXof9qjtgmPevsedTU/6l3zqHfLofXGj9omPuqbGhzkDCRDPDyD/6cpqCcmJaekpqWrc6Up6KgngiiwEJQBpWDcxOSkZAgzMSkpGcIsTcnJK03NLVAHAA "APL (Dyalog Extended) – Try It Online"
# [Python 3] REPL, 25 bytes _[~(len(_)%2)::-2]+_[::2] [Try it online!][TIO-khqsf6k8] ## Explanation _[~(len(_)%2)::-2]+_[::2] _[ :: ] substring -2 of every other item, backwards, from len(_) the length %2 mapped even => 0 odd => 1 ~( ) mapped 0 => -1 (last element) 1 => -2 (second-last) + joined with _[:: ] substring 2 of every other item [Python 3]: https://docs.python.org/3/ [TIO-khqsf6k8]: https://tio.run/##fVE9b8IwEN3zK66WKmxBo0K3SLDRsV26URSF5EIuOLblOCos/eupHSLSMvQttnz3Pu5sLq7S6qUvsIQyO2Fq0UjeViilSCLwKK1uINcFAjVGW@fvjSGJqT@bTBVDkySFLaxhIMatkeT47FPNxFAlX3m@imkLNZACm6kj8uUCJCo@sAXMYTl6BuSedGc1SMa1ppGyo6TeC3GjUOlZ1ILSDt60wkkswFhSPtVms5ktwCvFcQz/qQXgGXOe/30L09TR6EfwsP41w@Q4upEKM0h0OOzQLyT6qvxI8GG7MZ@zl4kWxEmZzvGrKZ5zNA62769ba7WdGg8Ws9N1p7dvI3GLNTVOZcsY69PdNw95U/G4EknytNrP012SrPa9r4qeZXDwSywAoYQjG7/Sp45YV0jVYWPYDw "Python 3 – Try It Online"
# [Jelly], 7 bytes ŒœṚ;¥@/ [Try it online!][TIO-khpc4b88] [Jelly]: https://github.com/DennisMitchell/jelly [TIO-khpc4b88]: https://tio.run/##y0rNyan8///opKOTH@6cZX1oqYP@////S1Ny8kpTcwsA "Jelly – Try It Online" ## Explanation ŒœṚ;¥@/ Main monadic link Œœ Split into odd and even indices / Fold over @ Reverse arguments ¥ Dyad ( Ṛ Reverse [left argument] ; Join [with right argument] ¥ )
# [Runic Enchantments], 30 bytes /!?={-1lirl< \l2%?r R$l0)?;',$ [Try it online!][TIO-knop1sh7] [Runic Enchantments]: https://github.com/Draco18s/RunicEnchantments/tree/Console [TIO-knop1sh7]: https://tio.run/##KyrNy0z@/19f0d62WtcwJ7Mox4YrJsdI1b6IK0glx0DT3lpdR@X//1KFFIUchTyFUoVUhVyFAgA "Runic Enchantments – Try It Online" Or if just squashing the inputs together with no joiner is allowed: # [Runic Enchantments], 17 bytes lril1-{)6*?l2%?r@ [Try it online!][TIO-knop5nd4] [Runic Enchantments]: https://github.com/Draco18s/RunicEnchantments/tree/Console [TIO-knop5nd4]: https://tio.run/##KyrNy0z@/z@nKDPHULda00zLPsdI1b7I4f//UoUUhRyFPIVShVSFXIUCAA "Runic Enchantments – Try It Online" In order for Runic to "read all input" the natively results in a pendulum encoding of said inputs.[^1] The only fiddly bit is whether the stack is in *forward* order or *reverse* order, depending on if there were an even or odd number of values in the input. In general, nothing special going on here. Read-input loop followed by a length-is-even check to reverse (or not) the stack, then a print-with-joiner loop or print-all-and-terminate. [^1]: Because `i`nput results in `NOP` if the input buffer is empty, the only safe place to store the current length of the stack prior to `i` is the bottom of the stack (A `S`wap command would corrupt the stack if there was no input to read!). Both `}` and `r` could be used, but `r` results in the desired behavior.
# TeX, 91 bytes This has restricted input, only `[A-Za-z]` work, everything else is considered the end of the string. Input must be of the form `\iftrue,<string>\relax\fi.`. ```tex \def~#1{\catcode`#1"D\def}~..{}~||{\fi\ifcat}~;;{a\edef.}~,,#1{|#1;{.#1}~}~~~#1{|#1;{#1.},} ``` Complete script running all test cases (I've added `><` around the output to make it easier to see that it works as intended): ```tex \def~#1{\catcode`#1"D\def}~..{}~||{\fi\ifcat}~;;{a\edef.}~,,#1{|#1;{.#1}~}~~~#1{|#1;{#1.},} {\tt>}\iftrue,abcdefg\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,a\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,abcd\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,ab\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,abd\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,abacbc\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,aabbcc\relax\fi.{\tt<} \def.{} % reset state {\tt>}\iftrue,udlnuemp\relax\fi.{\tt<} \bye ``` Output: 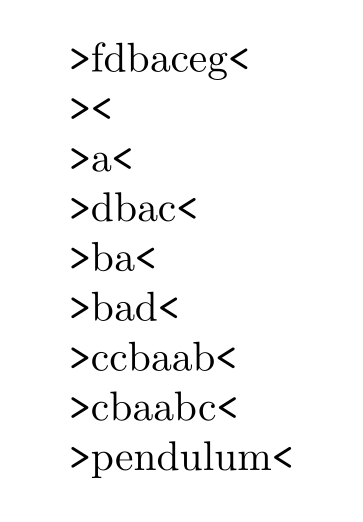