Jack Douglas
I want to style this simple HTML so that only one of the child divs is visible, but the parent remains a static size, sufficient for any one of the child divs, whichever one is visible at the time:
```htmlmixed
<div id="parent">
<div class="child">
</div>
<div class="child">
</div>
</div>
```
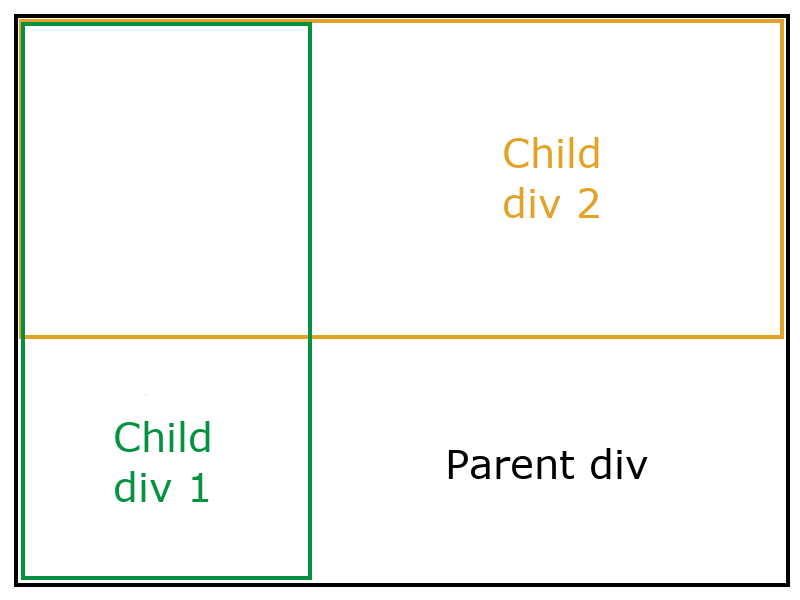
The illustration above has two child divs but there might be three or more.
How can I do this without JavaScript?
Top Answer
James Douglas
Using CSS grid:
You need to apply `display: inline-grid;` and `grid-template: max-content / max-content;` to the container element, and `grid-area: 1 / 1 / 2 / 2;` to each child element.
I use `inline-grid` rather than `grid` because it doesn't default to 100% width, but you can use either.
Unlike my other answer, this solution works for any number of child elements.
```css
#parent {
display: inline-grid;
grid-template: max-content / max-content;
border: 1px solid black;
}
#parent > div:first-child {
width: 100px;
height: 200px;
background: rgba(255, 0, 0, 0.5);
grid-area: 1 / 1 / 2 / 2;
}
#parent > div:last-child {
width: 200px;
height: 100px;
background: rgba(0, 0, 255, 0.5);
grid-area: 1 / 1 / 2 / 2;
}
```
This is what the CSS produces:
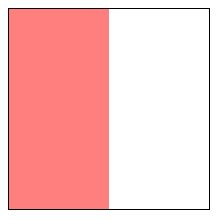
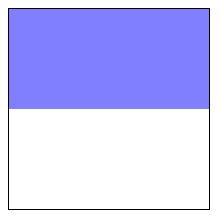
Here's a JSFiddle: https://jsfiddle.net/szd7rew1/
Answer #2
James Douglas
Using `float`:
You need to apply `float: left;` to the first child, and use `visibility: hidden;` or `opacity: 0;` to hide whichever div needs to be hidden.
**This solution only works if there are only two child divs**
```css
#parent {
display: inline-block;
border: 1px solid black;
}
#parent > div:first-child {
width: 100px;
height: 200px;
background: rgba(255, 0, 0, 0.5);
float: left;
}
#parent > div:last-child {
width: 200px;
height: 100px;
background: rgba(0, 0, 255, 0.5);
}
```
This is what the CSS produces, with one of the divs hidden using `visibility`:
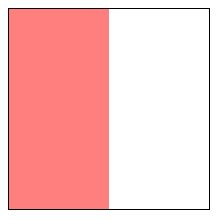
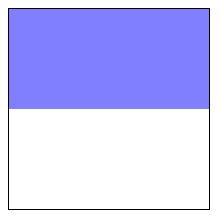
Here's a JSFiddle: https://jsfiddle.net/r1nLt8pj/