buster blue
This list would be made of the results of multiplying any 2 integers in the range, including 2 of the same integer. The order of the output list doesn't matter, nor does the presence of duplicate integers.
For example, given a range of 1 to 3, the output should be something like `[1, 2, 3, 4, 6, 9]`.
Top Answer
johnson
```
import itertools
[x*y for x, y in itertools.combinations_with_replacement([1,2,3], 2)]
```
Answer #2
Pax
Using list comprehension.
```python
def get_products(minFactor, maxFactor):
"""Assumes first parameter < 2nd parameter."""
products = [
multiplicand*multiplier
for multiplicand in range(minFactor, maxFactor+1)
for multiplier in range(multiplicand, maxFactor+1)
]
return products
```
Try in [repl.it](https://repl.it/@paxcodes/pythonta1143) --- along with other ways to do it.
This will return without duplicates---just so it's easier to verify the correctness of the output.
Otherwise, replace the 2nd for loop's range to `range(minFactor, maxFactor+1)`.
# References
[docs.python.org --- List Comprehensions](https://docs.python.org/3/tutorial/datastructures.html#list-comprehensions)
Answer #3
PeterVandivier
Using only builtins, you can do something like the following
::: tio lZHNToUwEIX3fYoxLijKT27uzoSFb@DemKaGgr3eDk0Lhj49DgWEG@PC2Uwy3@mZ4WBD/9Hhebq/KwfvyneNpcIvsHHMmDa2cz1I11rpvGIsNgfVz6h4du1gFPYvkfB0lRSyroVcGU/yMfmLBCKsVg0Y@akEDsbzMQvpEwMqb7rrPKONmtRGY4RphFdyOUI5HqAJtMTJQPD1LU6azoEmJTiJreKbdbb7PMJp3bvpL//Qz4XzLfAAl5upbghg189m2123744XF9JahTXH5UOc6ge3v6Of0oAQKI0SAqoKEiGM1ChEsljScZ6uWMOObY7b81@57IHPvBiz2MKisy5muqpTNlHlI5ymPMD5Gw
§§§ python python3
#!/usr/bin/env python
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-x')
parser.add_argument('-y')
def make_nums(x,y):
smol_num = int(min(x,y))
larg_num = int(max(x,y))
my_array = []
for i in range(smol_num, larg_num + 1):
for j in range(smol_num, larg_num + 1):
n = i * j
if n not in my_array:
my_array.append(n)
return my_array
if __name__ == '__main__':
args = parser.parse_args()
my_array = make_nums(args.x,args.y)
print(my_array)
§§§
:::
If the above code exists on your desktop as `ta.1143.py`, you might execute it as below...
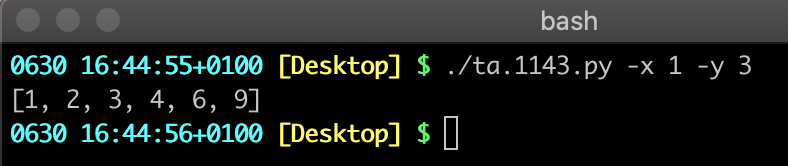