kus
I made a small application in blazor to evaluate asp.net core but long story short I ran into a bug / knowledge gap where I am unable to run a select query
https://dbfiddle.uk/?rdbms=postgres_14&fiddle=c693803f83e1c77ed8f96654f1e8b308
this is my insert statement
```sql
insert into myprogram (parentid, title, summary, description, owner, createdby, modifiedby) values
(0,'my title KQUS ZQVA KPUW MRZS SFJP PUKP WRPT KOFS SJZN 1','my summary 1',
'my description 1', 3,3, 3);
```
when I run this select
```sql
select
program.*,
owner.alias as ownername,
createdby.alias as createdbyname,
modifiedby.alias as modifiedbyname
from myprogram program
left join myperson owner on owner.id = program.owner
left join myperson createdby on createdby.id = program.createdby
left join myperson modifiedby on modifiedby.id = program.modifiedby
where program.title like concat('%','my title','%')
limit 200
```
I get results as expected but when I run this select I have no results
```sql
select
program.*,
owner.alias as ownername,
createdby.alias as createdbyname,
modifiedby.alias as modifiedbyname
from myprogram program
left join myperson owner on owner.id = program.owner
left join myperson createdby on createdby.id = program.createdby
left join myperson modifiedby on modifiedby.id = program.modifiedby
where program.title like concat('%','kqus','%')
limit 200
```
I tried to simplify the query but still no results
```sql
select
program.*
from myprogram program
where program.title like concat('%','kqus','%')
limit 200
```
What am I doing wrong here?
here is my csharp code (also at https://github.com/patstha/chappyblazor )
```
public async Task<IEnumerable<MyProgram>> SearchProgramsByTitle(string query)
{
query = query.ToUpper();
using NpgsqlConnection connection = new(_connectionString);
Stopwatch stopwatch = Stopwatch.StartNew();
IEnumerable<MyProgram> programs = await connection.QueryAsync<MyProgram>(
@"select
program.*,
owner.alias as ownername,
createdby.alias as createdbyname,
modifiedby.alias as modifiedbyname
from myprogram program
left join myperson owner on owner.id = program.owner
left join myperson createdby on createdby.id = program.createdby
left join myperson modifiedby on modifiedby.id = program.modifiedby
where uper(program.title) like concat('%',@query,'%')
limit 200
;", new { query = @query });
stopwatch.Stop();
foreach (MyProgram program in programs)
{
program.Stopwatch = stopwatch;
}
_logger.LogDebug("{methodName} returned {result}", nameof(GetPrograms), JsonConvert.SerializeObject(programs));
return programs;
}
```
PostgresException: 42883: function uper(character varying) does not exist
POSITION: 429
Npgsql.Internal.NpgsqlConnector.<ReadMessage>g__ReadMessageLong|211_0(NpgsqlConnector connector, bool async, DataRowLoadingMode dataRowLoadingMode, bool readingNotifications, bool isReadingPrependedMessage)
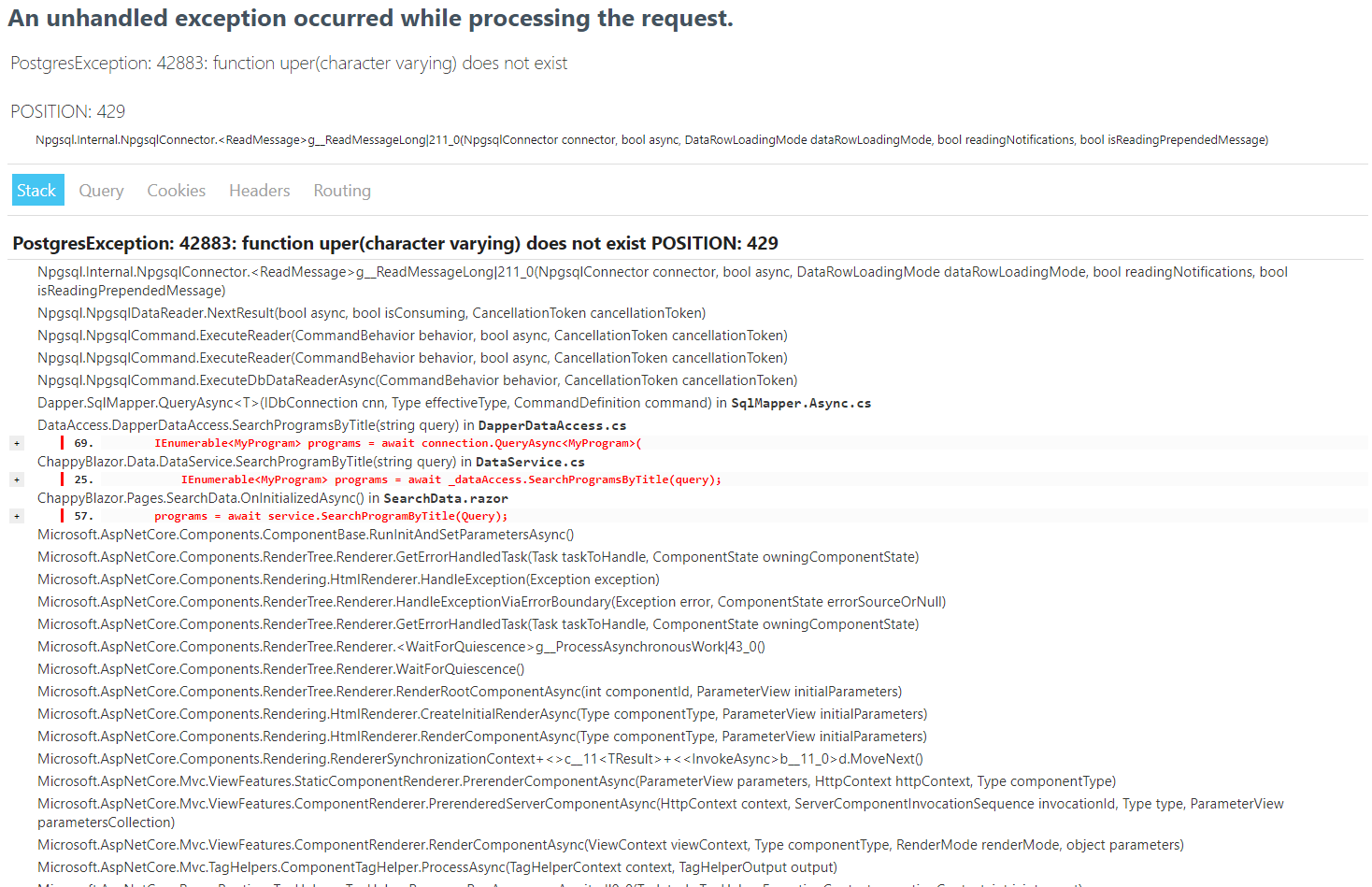
but I don't understand this error because I can see results when I query directly on elephantsql browser
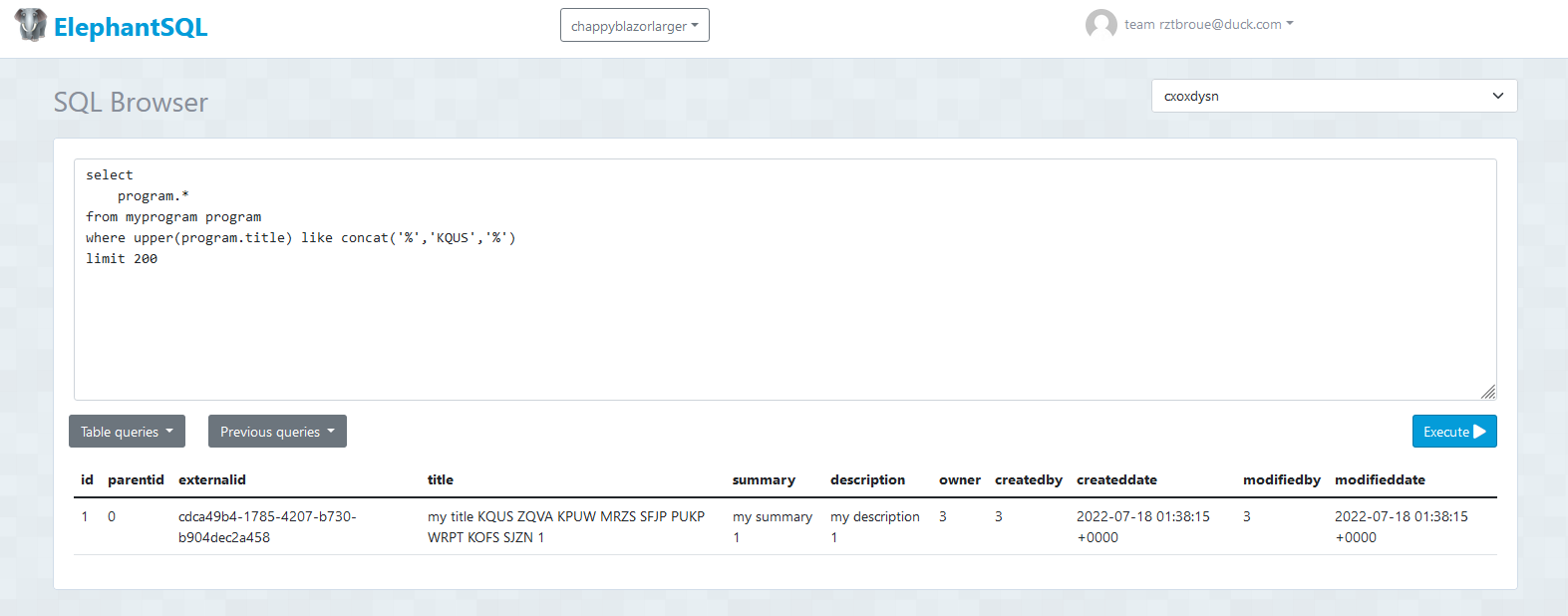
I can add more details if necessary :)
Top Answer
Jack Douglas
The db<>fiddle search string (`like concat('%','kqus','%')`)is in lowercase and the elephantsql search is in uppercase (`like concat('%','KQUS','%')`), matching the data in the table.
If you want a case insensitive `like`, you can use [`ilike`](https://www.postgresql.org/docs/14/functions-matching.html). Your fiddle [returns a result](https://dbfiddle.uk/?rdbms=postgres_14&fiddle=71b476f051613069c5d8decc1dc24c5e) with `ilike`.
> The key word ILIKE can be used instead of LIKE to make the match case-insensitive according to the active locale. This is not in the SQL standard but is a PostgreSQL extension.